Imagine you’re at a car dealership. You request a vehicle—say, a sedan or an SUV—and the factory builds it for you. You don’t need to know the intricate details of how the car is assembled; you just specify what you want, and the factory delivers. In software, the Factory Pattern operates similarly: it lets you create objects without directly specifying their exact classes. Instead, a factory handles the creation process, making your code more modular and adaptable.
In this post, we’ll explore:
- What the Factory Pattern is and why it matters
- How it works, with a simple shape-drawing example
- A real-world application in a logging system
- Its pros and cons
- Connections to other design patterns
- A Python implementation and its relevance in Python’s ecosystem
Let’s get started!
List blog-related:
- Basic Design Pattern – P1: Understanding the Factory Pattern
- Basic Design Pattern – P2: Mastering the Singleton Pattern
- Basic Design Pattern – P3: Exploring the Repository Pattern
- Basic Design Pattern – P4: Understanding the Builder Pattern
- Basic Design Pattern – P5: Mastering Dependency Injection
- Basic Design Pattern – P6: Understanding the Observer Pattern
- Basic Design Pattern – Final : A Summary and Comparison of Six Essential Patterns
What Is the Factory Pattern?
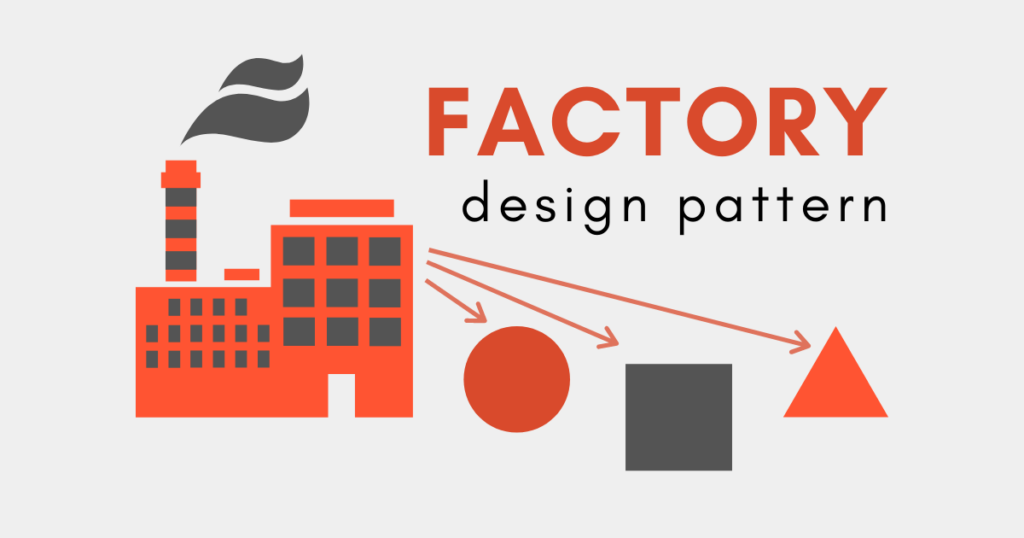
The Factory Pattern is all about decoupling object creation from the code that uses those objects. Normally, you might instantiate objects directly, like new Circle()
or new Square()
. While this works, it ties your code to specific classes, making it harder to modify or extend later.
With the Factory Pattern, a factory—a dedicated class or method—takes over the responsibility of creating objects. Your code interacts with the factory and an interface, not the concrete classes themselves. This separation offers:
- Flexibility: Swap out object types without changing the client code.
- Extensibility: Add new types easily by updating the factory.
- Open/Closed Principle: Keep your code open for extension but closed for modification.
In short, the Factory Pattern makes your codebase more modular and easier to maintain—a win for any project!
How Does the Factory Pattern Work?
The Factory Pattern involves a few key components:
- Product Interface: Defines the contract all objects must follow.
- Concrete Products: Specific classes implementing the interface.
- Factory: Creates and returns the appropriate object based on input.
Let’s see this in action with a simple shape-drawing application. We want to draw shapes like circles and squares without hardcoding their creation in the client code.
Example in Java
Step 1: Define the Product Interface
public interface Shape {
void draw();
}
Step 2: Create Concrete Products
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a circle");
}
}
public class Square implements Shape {
@Override
public void draw() {
System.out.println("Drawing a square");
}
}
Step 3: Build the Factory
public class ShapeFactory {
public Shape getShape(String shapeType) {
if (shapeType == null) {
return null;
}
if (shapeType.equalsIgnoreCase("CIRCLE")) {
return new Circle();
} else if (shapeType.equalsIgnoreCase("SQUARE")) {
return new Square();
}
return null;
}
}
Step 4: Use the Factory
public class Client {
public static void main(String[] args) {
ShapeFactory factory = new ShapeFactory();
Shape shape = factory.getShape("CIRCLE");
shape.draw(); // Output: Drawing a circle
}
}
Here, the ShapeFactory
decides which shape to instantiate based on the input string. The client only knows about the Shape
interface and the factory, making it easy to add new shapes (like a Triangle
) later without altering the client code.
Real-World Example: A Logging System
Let’s apply the Factory Pattern to a practical scenario: a logging system. In an application, you might need to log messages to a file, the console, or even a database, depending on your configuration. Without a factory, you’d end up with conditional logic everywhere, cluttering your code. The Factory Pattern centralizes this decision-making.
Implementation in Java
Product Interface
public interface Logger {
void log(String message);
}
Concrete Products
public class FileLogger implements Logger {
@Override
public void log(String message) {
System.out.println("Logged to file: " + message);
}
}
public class ConsoleLogger implements Logger {
@Override
public void log(String message) {
System.out.println("Logged to console: " + message);
}
}
Factory
public class LoggerFactory {
public Logger getLogger(String loggerType) {
if (loggerType.equalsIgnoreCase("FILE")) {
return new FileLogger();
} else if (loggerType.equalsIgnoreCase("CONSOLE")) {
return new ConsoleLogger();
}
return null;
}
}
Client Usage
public class Application {
public static void main(String[] args) {
String loggerType = "CONSOLE"; // Could come from config
LoggerFactory factory = new LoggerFactory();
Logger logger = factory.getLogger(loggerType);
logger.log("User logged in"); // Output: Logged to console: User logged in
}
}
Switching from console to file logging is as simple as changing loggerType
. The client code stays clean and focused, while the factory handles the complexity.
Advantages and Disadvantages
Advantages
- Decoupling: Client code depends on interfaces, not concrete classes.
- Extensibility: Add new types (e.g., a
DatabaseLogger
) without modifying existing code. - Single Responsibility: Creation logic lives in the factory, keeping other classes focused.
Disadvantages
- Complexity: Adds overhead, which might be unnecessary for small projects.
- Class Proliferation: Each new type requires a new class, potentially bloating the codebase.
The Factory Pattern shines in systems where object types might evolve, but it’s overkill for static, simple setups.
Related Patterns
The Factory Pattern doesn’t exist in isolation. It connects to other design patterns we’ll explore in this series:
- Singleton Pattern: A factory could be a singleton to ensure one instance manages creation.
- Builder Pattern: Focuses on constructing complex objects step-by-step, complementing the Factory Pattern.
- Dependency Injection: Factories often provide dependencies dynamically, aligning with DI principles.
Keep an eye out for these upcoming posts!
Factory Pattern in Python
Python’s dynamic nature might make you wonder: Do we need the Factory Pattern? While Python offers alternatives (like dictionaries for type mapping), the pattern still adds structure and clarity, especially in larger projects. Let’s adapt our logging system to Python.
Python Implementation
from abc import ABC, abstractmethod
# Product Interface
class Logger(ABC):
@abstractmethod
def log(self, message: str) -> None:
pass
# Concrete Products
class FileLogger(Logger):
def log(self, message: str) -> None:
print(f"Logged to file: {message}")
class ConsoleLogger(Logger):
def log(self, message: str) -> None:
print(f"Logged to console: {message}")
# Factory
class LoggerFactory:
def get_logger(self, logger_type: str) -> Logger:
if logger_type.upper() == "FILE":
return FileLogger()
elif logger_type.upper() == "CONSOLE":
return ConsoleLogger()
else:
raise ValueError(f"Unknown logger type: {logger_type}")
# Client Usage
def main():
logger_type = "CONSOLE" # Could come from config
factory = LoggerFactory()
logger = factory.get_logger(logger_type)
logger.log("User logged in successfully") # Output: Logged to console: User logged in successfully
if __name__ == "__main__":
main()
Is It a Good Fit for Python?
Python’s flexibility (e.g., duck typing) might tempt you to skip the Factory Pattern. However, it offers:
- Readability: Clearly defines object creation logic.
- Extensibility: Simplifies adding new logger types.
- Testability: Makes mocking and dependency injection easier.
That said, for tiny scripts or static object creation, simpler approaches might suffice. In larger systems, though, the Factory Pattern keeps things organized and maintainable.
Conclusion
The Factory Pattern is a cornerstone of object-oriented design, empowering you to create objects flexibly while keeping your code clean and adaptable. In this post, we’ve covered:
- Its core concept and benefits
- A simple shape-drawing example
- A real-world logging system application
- Its strengths, weaknesses, and ties to other patterns
- A Python implementation and its place in Python development
Design patterns are tools, not mandates. Use the Factory Pattern when it simplifies your project, and always prioritize readable, practical code.
Next up in our series: the Singleton Pattern. Until then, experiment with the Factory Pattern in your projects and let us know how it goes. Happy coding!
Leave a Reply