Welcome to our design patterns series! In this post, we’re exploring the Singleton Pattern, a creational design pattern that ensures a class has only one instance and provides a global point of access to it. Whether you’re managing configurations, handling database connections, or coordinating system-wide actions, the Singleton Pattern is a go-to solution when you need a single, shared instance.
If you’ve been following along, you might recall our previous discussion on the Factory Pattern (link to Factory Pattern post), which focuses on flexible object creation. Today, we’ll dive into the Singleton Pattern and show how it complements the Factory Pattern in certain scenarios.
In this guide, we’ll cover:
- What the Singleton Pattern is and why it’s useful
- How it works, with a detailed breakdown and code example
- Real-world examples to make it relatable
- Implementations in Java, Python, and C++
- Pros, cons, and best practices
- Its connection to the Factory Pattern
Let’s jump in!
List blog-related:
- Basic Design Pattern – P1: Understanding the Factory Pattern
- Basic Design Pattern – P2: Mastering the Singleton Pattern
- Basic Design Pattern – P3: Exploring the Repository Pattern
- Basic Design Pattern – P4: Understanding the Builder Pattern
- Basic Design Pattern – P5: Mastering Dependency Injection
- Basic Design Pattern – P6: Understanding the Observer Pattern
- Basic Design Pattern – Final : A Summary and Comparison of Six Essential Patterns
What Is the Singleton Pattern?
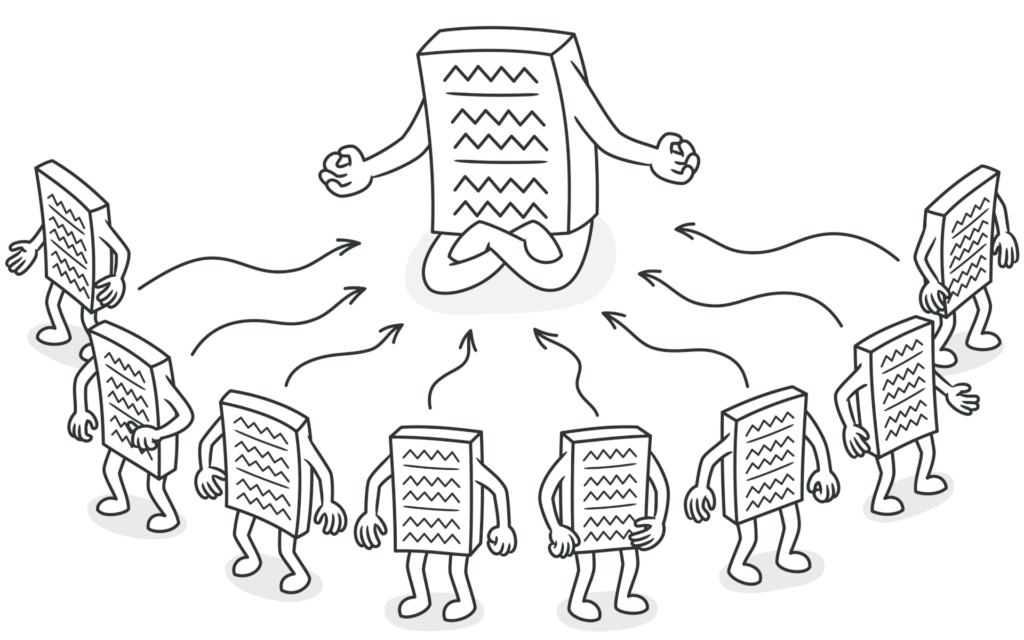
The Singleton Pattern is a creational design pattern that guarantees a class has exactly one instance and provides a global access point to that instance. Imagine it as a single key to a locked room—there’s only one, and everyone who needs access uses it.
This pattern shines in situations where you need a single object to manage shared resources or coordinate actions across an application. Common use cases include:
- A configuration manager storing application-wide settings.
- A logging system recording events consistently.
- A database connection pool optimizing resource usage.
Why Use It?
The Singleton Pattern offers:
- Efficiency: Prevents the creation of multiple resource-heavy objects.
- Consistency: Ensures all parts of the system use the same instance.
- Control: Centralizes access to a critical component.
It’s all about maintaining a single “source of truth” for a specific class.
How Does the Singleton Pattern Work?
The Singleton Pattern enforces its “one instance only” rule using a few key elements:
- Private Constructor: Blocks external instantiation with the
new
keyword. - Static Instance: Holds the single instance as a private static variable.
- Static Access Method: A public static method (e.g.,
getInstance()
) that:
- Checks if the instance exists (e.g., is it
null
?). - Creates it if it doesn’t exist (using the private constructor).
- Returns the existing instance on all subsequent calls.
This design ensures that every request for the instance returns the same object.
Code Example in Java
Here’s a simple Java implementation:
public class Singleton {
// The single instance, initially null
private static Singleton instance;
// Private constructor to prevent external instantiation
private Singleton() {
System.out.println("Singleton instance created!");
}
// Public method to access the instance
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton(); // Create the instance if it doesn’t exist
}
return instance; // Return the existing instance
}
// A sample method to test the instance
public void sayHello() {
System.out.println("Hello from the Singleton!");
}
}
// Test the Singleton
public class Main {
public static void main(String[] args) {
Singleton s1 = Singleton.getInstance();
Singleton s2 = Singleton.getInstance();
s1.sayHello(); // Output: Hello from the Singleton!
System.out.println(s1 == s2); // Output: true (same instance)
}
}
How It Works:
- The first
getInstance()
call creates the instance since it’snull
. - The second call returns the same instance without creating a new one.
- The
==
comparison confirmss1
ands2
are the same object.
Key Notes:
- Lazy Initialization: The instance is created only when needed, conserving resources.
- Thread Safety: In multi-threaded environments, you might need
synchronized
to avoid race conditions (e.g.,public static synchronized Singleton getInstance()
).
Implementation in Different Languages
The Singleton Pattern adapts to various programming languages. Here are implementations in Java, Python, and C++.
Java Implementation
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static synchronized Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
public void showMessage() {
System.out.println("Hello from Singleton!");
}
}
Python Implementation
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
def show_message(self):
print("Hello from Singleton!")
C++ Implementation
#include <iostream>
class Singleton {
private:
static Singleton* instance;
Singleton() {} // Private constructor
public:
static Singleton* getInstance() {
if (instance == nullptr) {
instance = new Singleton();
}
return instance;
}
void showMessage() {
std::cout << "Hello from Singleton!" << std::endl;
}
};
Singleton* Singleton::instance = nullptr;
Each version ensures a single instance with a global access point, tailored to the language’s conventions.
Pros and Cons of the Singleton Pattern
The Singleton Pattern has its strengths and weaknesses.
Advantages
- Controlled Access: Prevents conflicts from multiple instances.
- Global Access: Simplifies reaching the instance from anywhere.
- Resource Efficiency: Optimizes usage of shared resources.
Disadvantages
- Global State: Introduces global state, which can complicate testing and debugging.
- Concurrency Issues: Requires extra care in multi-threaded applications.
- Overuse Risk: Can be misapplied where a single instance isn’t needed, adding unnecessary complexity.
Use it when a single instance is truly required—not as a catch-all solution.
Best Practices and Tips
To get the most out of the Singleton Pattern:
- Lazy Initialization: Create the instance only when requested.
- Thread Safety: Add synchronization or use language-specific features (e.g., Python’s
__new__
) for multi-threaded safety. - Use Judiciously: Reserve it for cases needing a single instance—avoid treating it like a global variable.
- Testability: Watch out for global state in tests; consider alternatives like dependency injection when feasible.
These practices ensure the pattern works for you, not against you.
Connection to the Factory Pattern
If you’ve explored our previous post on the Factory Pattern (link to Factory Pattern post), you’ll notice both are creational patterns, but they tackle different problems:
- The Factory Pattern creates objects flexibly without hardcoding their classes.
- The Singleton Pattern ensures only one instance exists and provides global access.
These patterns can complement each other. For example, a factory might return a singleton instance for certain objects, blending the factory’s flexibility with the singleton’s efficiency. This combination can streamline resource management while keeping object creation adaptable.
Conclusion
The Singleton Pattern is a vital tool in object-oriented design, ensuring a class has one instance and a global access point. In this post, we’ve covered:
- Its definition and purpose
- How it works, with a practical example
- Real-world analogies
- Implementations in Java, Python, and C++
- Its pros, cons, and best practices
- How it ties to the Factory Pattern
While powerful, the Singleton Pattern requires careful use to avoid pitfalls like global state. Apply it thoughtfully, and it’ll serve you well.
Next up: the Repository Pattern. For now, try implementing the Singleton Pattern in your projects and share your experiences. Happy coding!
Leave a Reply