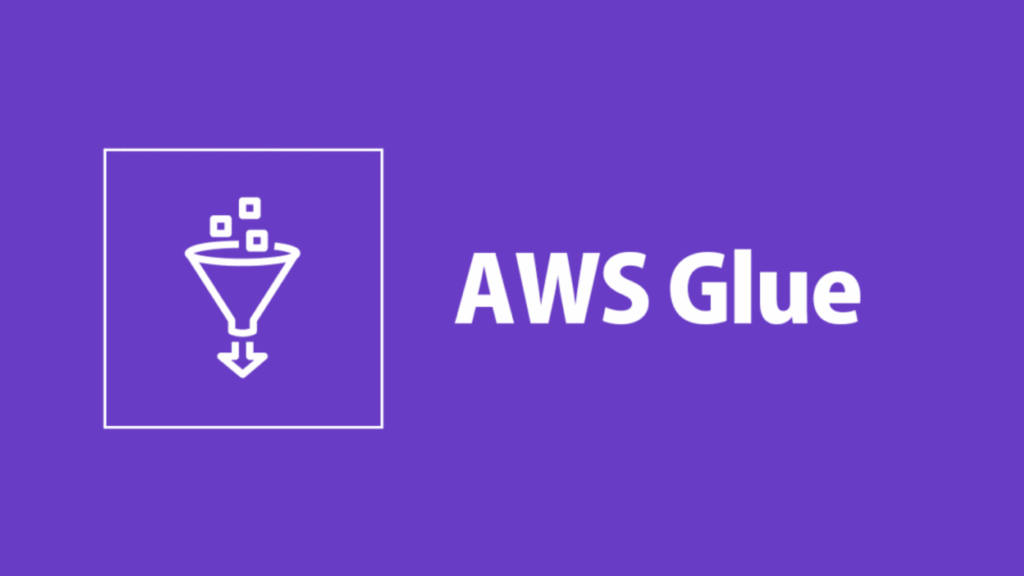
As a developer working with AWS Glue and Apache Spark, one of the most powerful tools in your performance optimization toolkit is caching. Caching can significantly reduce computation time and resource usage, especially in complex ETL (Extract, Transform, Load) pipelines where the same data is reused across multiple operations. In this blog, I’ll explain how caching works in AWS Glue Spark, why it’s efficient, and when to use it, using a practical example inspired by a typical Glue job. My goal is to make this concept clear and approachable for developers who are new to Spark or looking to optimize their Glue jobs.
What is Caching in Spark?
In Apache Spark, caching is the process of storing a DataFrame or RDD (Resilient Distributed Dataset) in memory (or disk) so that it can be reused across multiple operations without recomputing it. Spark’s lazy evaluation means that transformations (like filters, joins, or window functions) are not executed immediately. Instead, they build a logical execution plan. Only when an action (like writing to S3, creating a view, or collecting results) is triggered does Spark compute the data.
Without caching, if a DataFrame is used in multiple parts of your job, Spark will recompute it each time it’s needed. This can be expensive, especially if the DataFrame is derived from complex transformations or involves reading data from external storage like Amazon S3. Caching allows you to compute the DataFrame once, store it in memory, and reuse it, saving both time and resources.
A Real-World Example: Why Caching Matters
Let’s walk through a simplified AWS Glue Spark job to illustrate how caching works and why it’s efficient. Imagine you’re processing student data to generate a report. You have a DataFrame called stu_latest
, which contains the latest student records after applying some expensive transformations, such as window functions to deduplicate records. This DataFrame is used in two parts of your pipeline:
- To join with course data to create a report on student-course enrollment.
- To detect spoofed student records based on some logic.
Here’s a sample Glue job code snippet to set the stage:
from pyspark.sql import SparkSession
from pyspark.sql.functions import col, row_number
from pyspark.sql.window import Window
# Initialize Spark session
spark = SparkSession.builder.appName("StudentETL").getOrCreate()
# Read student data from S3
students_df = spark.read.parquet("s3://my-bucket/students/")
# Apply window function to get the latest student record per ID
window_spec = Window.partitionBy("student_id").orderBy(col("timestamp").desc())
stu_latest = students_df.withColumn("rn", row_number().over(window_spec)).filter(col("rn") == 1).drop("rn")
# Cache stu_latest
stu_latest.cache()
# Path 1: Join with course data
course_stu = stu_latest.join(courses_df, "student_id")
bc_stu_course = course_stu.groupBy("course_id").agg({"student_id": "count"})
bc_user_course = bc_stu_course.join(users_df, "course_id")
bc_user_course_stu = bc_user_course.join(stu_latest, "student_id")
# Path 2: Detect spoofed students
stu_spoofed = stu_latest.filter(col("is_spoofed") == True)
# Combine results
final_df = bc_user_course_stu.union(stu_spoofed.select(bc_user_course_stu.columns))
# Action: Create a temporary view (triggers computation)
final_df.createOrReplaceTempView("new_data")
# Write final output to S3
final_df.write.parquet("s3://my-bucket/output/")
In this example, stu_latest
is a critical DataFrame that’s used in two execution paths:
- Path 1: Joins and aggregations to create a student-course report.
- Path 2: Filtering to identify spoofed student records.
Let’s break down what happens when this code runs, focusing on when stu_latest
is computed and why caching it is efficient.
When is stu_latest
Computed?
Spark’s lazy evaluation means that transformations like withColumn
, filter
, and join
don’t compute stu_latest
immediately. Instead, Spark builds a directed acyclic graph (DAG) of transformations. The computation of stu_latest
is triggered only when an action requires its data.
In our example, the first action that triggers computation is:
final_df.createOrReplaceTempView("new_data")
This action forces Spark to compute final_df
, which depends on bc_user_course_stu
and stu_spoofed
, both of which depend on stu_latest
. Spark traces the dependency chain backward and computes stu_latest
as part of the execution plan.
Without caching, Spark would compute stu_latest
twice:
- Once for the course-related path (
bc_user_course_stu
). - Once for the spoofed records path (
stu_spoofed
).
This is inefficient because stu_latest
is derived from an expensive window function that involves:
- Reading data from S3 (I/O-intensive).
- Partitioning and sorting data across the cluster (CPU-intensive).
By calling stu_latest.cache()
, you instruct Spark to:
- Compute
stu_latest
once when the first action triggers it. - Store the result in memory (or disk, if memory is full).
- Reuse the cached version for all subsequent operations.
The Dependency Chain: Visualizing the Reuse
To understand why caching stu_latest
is efficient, let’s trace the dependency chain:
stu_latest
├── Path 1: Course Data
│ ├── stu_with_arrays (join with courses)
│ ├── course_stu
│ ├── bc_stu_course (group by)
│ ├── bc_user_course (join with users)
│ ├── bc_user_course_stu (join with stu_latest again)
│ └── final_df
└── Path 2: Spoofed Detection
├── stu_spoofed (filter)
└── final_df
When final_df.createOrReplaceTempView("new_data")
executes:
- Spark computes the entire dependency chain.
stu_latest
is needed in both Path 1 (for joins and aggregations) and Path 2 (for filtering spoofed records).- Without caching, the window function for
stu_latest
would run twice, duplicating I/O and CPU work. - With caching,
stu_latest
is computed once, stored in memory, and reused for both paths.
Why is Caching Efficient?
Caching stu_latest
is efficient for several reasons:
- Reused Across Multiple Paths:
stu_latest
is used in two execution paths. Caching ensures it’s computed only once, avoiding redundant calculations. - Expensive Transformations: The window function to create
stu_latest
involves partitioning and sorting, which are resource-intensive. Caching prevents Spark from repeating this work. - Reduced I/O Overhead: Reading data from S3 is slow compared to accessing in-memory data. Caching
stu_latest
eliminates multiple S3 reads. - Minimal Memory Trade-Off: While caching consumes memory, the performance gain from avoiding recomputation often outweighs the memory cost. In AWS Glue, you can monitor memory usage and adjust the worker type (e.g., G.1X or G.2X) or number of workers to accommodate caching.
- Scalability: In large-scale ETL jobs, where datasets are in the terabyte range, caching intermediate results like
stu_latest
can save hours of processing time.
When Should You Cache in AWS Glue?
Caching is not always the answer, as it consumes memory and may not be necessary for small datasets or simple transformations. Here are some guidelines for when to cache in AWS Glue:
- Cache Reused DataFrames: If a DataFrame is used multiple times in your job (e.g., in multiple joins, filters, or aggregations), caching it can save significant time.
- Cache Expensive Computations: DataFrames derived from complex operations like window functions, large joins, or heavy aggregations are good candidates for caching.
- Monitor Memory Usage: In AWS Glue, use the Spark UI or CloudWatch metrics to ensure caching doesn’t cause memory spills to disk, which can negate performance gains.
- Unpersist When Done: If a cached DataFrame is no longer needed, call
unpersist()
to free up memory. For example:
stu_latest.unpersist()
- Consider Data Size: For very large DataFrames, caching may not fit in memory. In such cases, consider persisting to disk with
stu_latest.persist(StorageLevel.DISK_ONLY)
or optimizing your job to reduce the DataFrame’s size before caching.
Common Misconceptions About Caching
One common misunderstanding is that caching is unnecessary if all operations are transformations, since transformations are lazily evaluated. While it’s true that transformations only build the execution plan, the plan’s execution (triggered by an action) may involve recomputing the same DataFrame multiple times if it’s not cached. In our example, even though stu_latest
is defined by transformations, its reuse in multiple paths means caching saves significant computation.
Another misconception is that caching always improves performance. If a DataFrame is used only once or is very small, caching may add overhead without benefits. Always analyze your job’s DAG (via the Spark UI) to decide whether caching is worthwhile.
Best Practices for Caching in AWS Glue
To make the most of caching in your Glue jobs:
- Profile Your Job: Use the Spark UI to identify DataFrames that are recomputed multiple times. The DAG visualization can show you where caching will have the most impact.
- Cache Strategically: Cache DataFrames that are reused or expensive to compute, but avoid caching every intermediate result.
- Tune Glue Resources: Ensure your Glue job has enough memory (e.g., use G.2X workers for larger datasets) to support caching without spilling to disk.
- Test Incrementally: Start with caching one or two key DataFrames, measure the performance impact, and adjust as needed.
- Clean Up: Unpersist cached DataFrames when they’re no longer needed to free up memory for other operations.
Conclusion
Caching in AWS Glue Spark is a powerful technique for optimizing ETL jobs, especially when dealing with complex transformations and reused DataFrames. By storing intermediate results like stu_latest
in memory, you can avoid redundant computations, reduce I/O overhead, and significantly speed up your jobs. The key is to understand your job’s dependency chain, identify reused or expensive DataFrames, and cache strategically.
In our example, caching stu_latest
ensured that an expensive window function was computed only once, even though the DataFrame was used in multiple execution paths. This approach is particularly valuable in AWS Glue, where I/O operations (like reading from S3) and distributed computations can be costly.
As you build your Glue jobs, experiment with caching, monitor performance via the Spark UI, and fine-tune your resource allocation. With these practices, you’ll be well-equipped to write efficient, scalable ETL pipelines that make the most of Spark’s capabilities.
Happy coding, and may your Glue jobs run faster than ever!
Leave a Reply