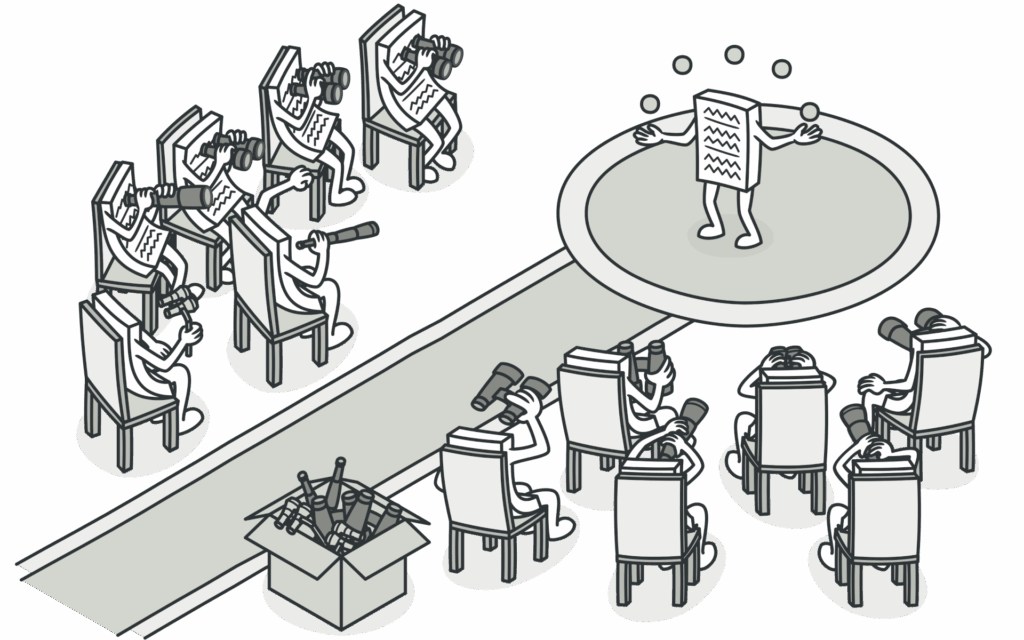
Welcome to the final installment of our design patterns series! Today, we’re exploring the Observer Pattern, a behavioral design pattern that’s essential for building responsive, event-driven systems. If you’ve ever wondered how GUIs update instantly when you click a button or how notifications work in apps, the Observer Pattern is likely at play behind the scenes.
In this post, we’ll cover:
- What the Observer Pattern is and why it’s so useful
- How it works, with a simple analogy and code example
- Real-world applications to see it in action
- Its pros and cons
- How it connects to other patterns we’ve discussed
- A look back at the series and what’s next
Let’s dive in!
What Is the Observer Pattern?
The Observer Pattern is a design pattern where an object, called the subject, maintains a list of its dependents, called observers, and automatically notifies them of any state changes. It’s like a newsletter: the newsletter (subject) has subscribers (observers), and whenever a new issue is published, all subscribers are notified.
This pattern is perfect for scenarios where one part of your system needs to react to changes in another part, without tightly coupling them.
Key Components:
- Subject: The object being observed. It keeps a list of observers and notifies them when its state changes.
- Observers: Objects that need to be updated when the subject changes. They register with the subject to receive notifications.
The Observer Pattern promotes loose coupling—the subject doesn’t need to know the details of its observers, and vice versa.
How Does the Observer Pattern Work?
Here’s the step-by-step process:
- Registration: Observers register with the subject to receive updates.
- State Change: When the subject’s state changes (e.g., new data arrives), it notifies all registered observers.
- Update: Each observer reacts to the notification by updating itself, often by pulling the new data from the subject.
This mechanism ensures that all interested parties stay in sync without the subject needing to know who they are or what they do.
Analogy: A Weather Station
Imagine a weather station (subject) that measures temperature. Multiple displays (observers)—like a phone app, a website, and a smartwatch—want to show the latest temperature. When the weather station records a new temperature, it notifies all displays, and they update accordingly.
This is the Observer Pattern in action: the weather station doesn’t care how many displays there are or what they do with the data; it just broadcasts the change.
Example Implementation
Let’s bring this to life with a simple Python example. We’ll create a WeatherStation
(subject) and two displays (observers): PhoneDisplay
and WatchDisplay
.
The Code
from abc import ABC, abstractmethod
# Observer Interface
class Observer(ABC):
@abstractmethod
def update(self, temperature: float) -> None:
pass
# Subject
class WeatherStation:
def __init__(self) -> None:
self._observers: list[Observer] = []
self._temperature: float = 0.0
def register_observer(self, observer: Observer) -> None:
self._observers.append(observer)
def remove_observer(self, observer: Observer) -> None:
self._observers.remove(observer)
def notify_observers(self) -> None:
for observer in self._observers:
observer.update(self._temperature)
def set_temperature(self, temperature: float) -> None:
self._temperature = temperature
self.notify_observers()
# Concrete Observers
class PhoneDisplay(Observer):
def update(self, temperature: float) -> None:
print(f"Phone display: Temperature updated to {temperature}°C")
class WatchDisplay(Observer):
def update(self, temperature: float) -> None:
print(f"Watch display: Temp is now {temperature}°C")
# Usage
station = WeatherStation()
phone = PhoneDisplay()
watch = WatchDisplay()
station.register_observer(phone)
station.register_observer(watch)
station.set_temperature(25.0)
# Output:
# Phone display: Temperature updated to 25.0°C
# Watch display: Temp is now 25.0°C
station.set_temperature(30.0)
# Output:
# Phone display: Temperature updated to 30.0°C
# Watch display: Temp is now 30.0°C
How It Works:
- The
WeatherStation
holds a list of observers and notifies them whenever the temperature changes. - The
PhoneDisplay
andWatchDisplay
implement theObserver
interface and define how they react to updates. - When
set_temperature
is called, the station notifies all registered observers, and they print the new temperature.
This setup makes it easy to add or remove displays without changing the weather station’s code.
Real-World Applications
The Observer Pattern is everywhere in modern software:
- GUI Frameworks: In frameworks like Java’s Swing or Python’s Tkinter, UI components (observers) update when the underlying data (subject) changes.
- Event Listeners: JavaScript’s event listeners (e.g.,
addEventListener
) use the Observer Pattern to handle user interactions. - Pub-Sub Systems: Messaging systems like Redis or Kafka use publish-subscribe (a variant of Observer) to distribute messages to subscribers.
- Reactive Programming: Libraries like RxJS or ReactiveX rely on the Observer Pattern to handle asynchronous data streams.
If you’ve ever built a UI or worked with events, you’ve likely used the Observer Pattern without realizing it.
Advantages and Disadvantages
Advantages
- Loose Coupling: The subject and observers are independent; they only interact through a simple interface.
- Scalability: Easily add or remove observers without modifying the subject.
- Reusability: Observers can be reused across different subjects, and vice versa.
Disadvantages
- Performance: If there are many observers, notifying them all can be slow.
- Complexity: In large systems, managing observer lifecycles (e.g., removing stale observers) can be tricky.
- Debugging: It can be harder to trace the flow of notifications in complex setups.
The Observer Pattern is ideal for systems where components need to react to changes dynamically, but it’s not a one-size-fits-all solution.
Connection to Other Patterns
Since this is the final topic in our series, let’s see how the Observer Pattern ties into the patterns we’ve already covered:
- Factory Pattern (link to Factory Pattern post): A factory can create observers dynamically based on runtime conditions.
- Singleton Pattern (link to Singleton Pattern post): The subject could be a singleton, ensuring there’s only one instance broadcasting updates.
- Repository Pattern (link to Repository Pattern post): A repository could notify observers when data changes, keeping the UI in sync.
- Builder Pattern (link to Builder Pattern post): Builders can construct complex observers or subjects step by step.
- Dependency Injection (link to DI post): DI can inject observers into subjects or manage their lifecycles.
For example, you might use a factory to create different types of observers and inject them into a singleton subject. These patterns often work together to build robust, flexible systems.
Conclusion and Series Wrap-Up
The Observer Pattern is a powerful tool for creating responsive, decoupled systems. In this post, we’ve explored:
- What the Observer Pattern is and how it works
- A simple weather station example
- Real-world applications in GUIs, event systems, and more
- Its strengths and weaknesses
- How it connects to other design patterns
This marks the end of our design patterns series, where we’ve journeyed through creational, structural, and behavioral patterns. Here’s a quick recap of what we’ve covered:
- Factory Pattern: Flexible object creation
- Singleton Pattern: Ensuring a single instance
- Repository Pattern: Abstracting data access
- Builder Pattern: Step-by-step object construction
- Dependency Injection: Managing dependencies
- Observer Pattern: Event-driven communication
Each pattern solves specific problems, and knowing when to use them is key to writing clean, maintainable code. I encourage you to experiment with these patterns in your projects—start small, and build up as you get comfortable.
Thank you for joining me on this journey! If you have questions or want to share your experiences, drop a comment below. Happy coding, and stay tuned for more insights in the future!
Leave a Reply