Welcome back to our design patterns series! Today, we’re diving into Dependency Injection (DI), a powerful technique that’s essential for writing flexible, testable, and maintainable code. If you’ve ever struggled with tightly coupled classes or wished for an easier way to manage dependencies, this post is for you.
In this guide, we’ll explore:
- What Dependency Injection is and why it’s a game-changer
- How it works, with a simple example
- A real-world scenario to see it in action
- Its pros and cons
- How it ties into other patterns we’ve covered
- What’s next in the series
Let’s get started!
List blog-related:
- Basic Design Pattern – P1: Understanding the Factory Pattern
- Basic Design Pattern – P2: Mastering the Singleton Pattern
- Basic Design Pattern – P3: Exploring the Repository Pattern
- Basic Design Pattern – P4: Understanding the Builder Pattern
- Basic Design Pattern – P5: Mastering Dependency Injection
- Basic Design Pattern – P6: Understanding the Observer Pattern
- Basic Design Pattern – Final : A Summary and Comparison of Six Essential Patterns
What Is Dependency Injection?
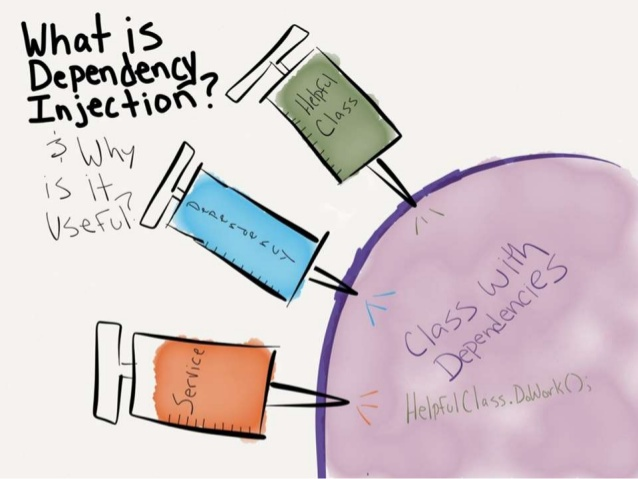
Dependency Injection is a design pattern that helps you manage the relationships between classes. Instead of a class creating its own dependencies (like other objects it needs to function), those dependencies are injected from the outside. This decouples your classes, making them more modular and easier to test.
Think of it like ordering a coffee: you don’t need to know how the coffee machine works or where the beans come from—you just ask for a coffee, and it’s handed to you. In DI, your class asks for what it needs, and the injector provides it.
Key Ideas:
- Decoupling: Classes don’t create their own dependencies; they receive them.
- Flexibility: Swap out implementations without changing the class itself.
- Testability: Easily inject mock objects for testing.
In short, DI makes your code more adaptable and less brittle.
How Does Dependency Injection Work?
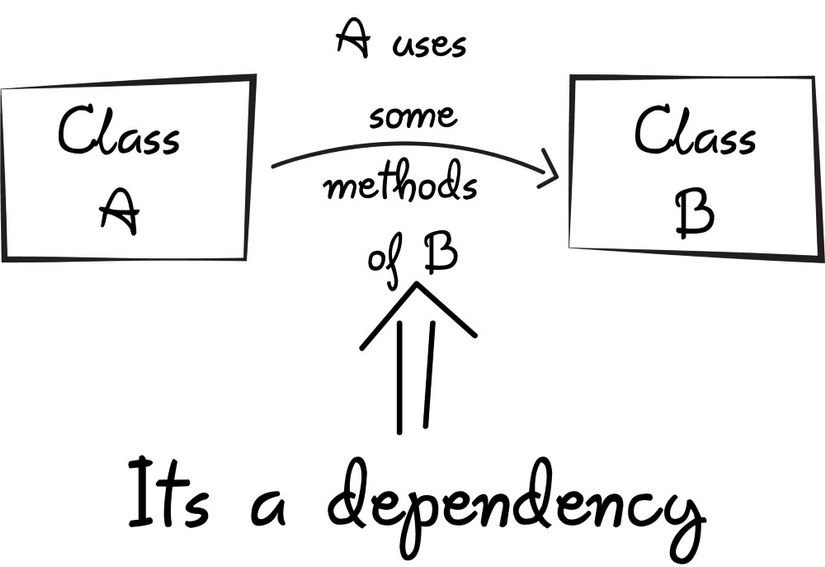
There are three main ways to inject dependencies:
- Constructor Injection: Dependencies are passed through the constructor.
- Setter Injection: Dependencies are set via setter methods.
- Interface Injection: Dependencies are injected through an interface method.
The most common approach is constructor injection, as it ensures the class has everything it needs from the start. Let’s see it in action with a simple example.
Example: A Notification Service
Imagine you’re building a notification system that can send messages via email or SMS. Without DI, you might hardcode the email service inside the notification class. But with DI, you can inject the service, making it easy to switch between email and SMS.
The Code (in Python)
# The Dependency (Service Interface)
class MessageService:
def send(self, message: str) -> None:
pass
# Concrete Implementations
class EmailService(MessageService):
def send(self, message: str) -> None:
print(f"Sending email: {message}")
class SMSService(MessageService):
def send(self, message: str) -> None:
print(f"Sending SMS: {message}")
# The Client Class
class Notification:
def __init__(self, service: MessageService) -> None:
self.service = service
def notify(self, message: str) -> None:
self.service.send(message)
# Usage
email_service = EmailService()
notification = Notification(email_service)
notification.notify("Hello via email!") # Output: Sending email: Hello via email!
sms_service = SMSService()
notification_with_sms = Notification(sms_service)
notification_with_sms.notify("Hello via SMS!") # Output: Sending SMS: Hello via SMS!
How It Works:
- The
Notification
class doesn’t care how the message is sent; it just needs aMessageService
. - You can inject any service that implements
MessageService
, likeEmailService
orSMSService
. - This makes it easy to add new services (e.g.,
PushNotificationService
) without changingNotification
.
This is DI’s magic: it keeps your classes focused and adaptable.
Real-World Example: A Web Application
Let’s apply DI to a more realistic scenario: a web app that needs to fetch user data from a database. Instead of hardcoding the database connection inside the user service, we’ll inject it.
The Code (in Python)
# The Dependency (Repository Interface)
class UserRepository:
def get_user(self, user_id: int) -> str:
pass
# Concrete Implementations
class DatabaseUserRepository(UserRepository):
def get_user(self, user_id: int) -> str:
# Simulate fetching from a database
return f"User {user_id} from database"
class MockUserRepository(UserRepository):
def get_user(self, user_id: int) -> str:
return f"Mock user {user_id}"
# The Client Class
class UserService:
def __init__(self, repository: UserRepository) -> None:
self.repository = repository
def get_user_name(self, user_id: int) -> str:
return self.repository.get_user(user_id)
# Usage in Production
db_repo = DatabaseUserRepository()
user_service = UserService(db_repo)
print(user_service.get_user_name(1)) # Output: User 1 from database
# Usage in Testing
mock_repo = MockUserRepository()
test_user_service = UserService(mock_repo)
print(test_user_service.get_user_name(1)) # Output: Mock user 1
Why It’s Powerful:
- Flexibility: Switch from a database to an API or in-memory store by changing the injected repository.
- Testability: Inject a mock repository to test
UserService
without hitting a real database. - Clean Code:
UserService
stays focused on business logic, not data access details.
This is DI in the wild, making your app more modular and testable.
Pros and Cons of Dependency Injection
Pros
- Decoupling: Classes are less dependent on specific implementations.
- Testability: Easily inject mocks or stubs for isolated testing.
- Flexibility: Swap dependencies without changing the client class.
- Maintainability: Changes in one part (e.g., data access) don’t ripple through the codebase.
Cons
- Complexity: Adds an extra layer, which can feel like overkill for small projects.
- Learning Curve: Requires understanding interfaces and abstraction.
- Boilerplate: You might write more code upfront (e.g., interfaces and injectors).
DI is ideal for medium to large projects where maintainability and testability matter. For tiny scripts, it might be unnecessary.
Connection to Other Patterns
Since this is part of our series, let’s link DI to patterns we’ve already covered:
- Factory Pattern (link to Factory Pattern post): A factory can create and inject dependencies dynamically.
- Singleton Pattern (link to Singleton Pattern post): DI can manage singletons, ensuring they’re injected where needed without global state.
- Repository Pattern (link to Repository Pattern post): DI is perfect for injecting repositories into services, as shown in the example.
For instance, a factory could inject a singleton repository into a service, blending multiple patterns for a robust architecture.
Conclusion and Next Steps
Dependency Injection is a cornerstone of modern software design, promoting loose coupling and testability. In this post, we’ve covered:
- What DI is and why it’s valuable
- A simple notification example
- A real-world web app scenario
- Its advantages and trade-offs
- How it connects to other patterns
Next up, we’ll explore the Observer Pattern, a behavioral pattern that’s perfect for event-driven systems. Until then, try applying DI in your projects and see how it transforms your code. Happy coding!
Leave a Reply