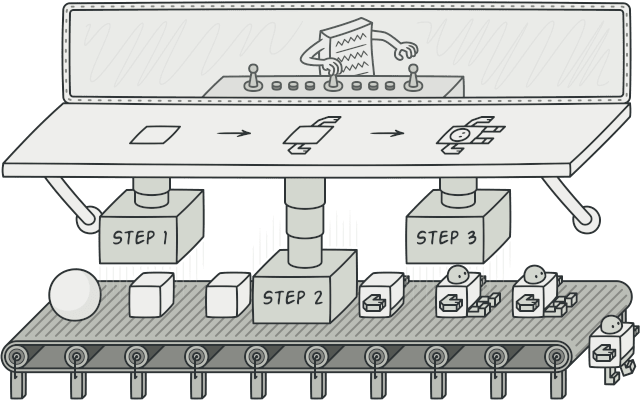
What Is the Builder Pattern?
The Builder Pattern is a creational design pattern that lets you build complex objects step by step. Instead of stuffing every possible parameter into a constructor (and ending up with a mess), it uses a separate builder object to handle the construction process. This keeps your code clean, flexible, and reusable.
Imagine ordering a custom pizza: you tell the chef to add cheese, then pepperoni, then mushrooms—one step at a time. The chef (the builder) assembles it exactly how you want. That’s the Builder Pattern in a nutshell: it separates how an object is built from what the final object looks like.
List blog-related:
- Basic Design Pattern – P1: Understanding the Factory Pattern
- Basic Design Pattern – P2: Mastering the Singleton Pattern
- Basic Design Pattern – P3: Exploring the Repository Pattern
- Basic Design Pattern – P4: Understanding the Builder Pattern
- Basic Design Pattern – P5: Mastering Dependency Injection
- Basic Design Pattern – P6: Understanding the Observer Pattern
- Basic Design Pattern – Final : A Summary and Comparison of Six Essential Patterns
The Concept Behind It
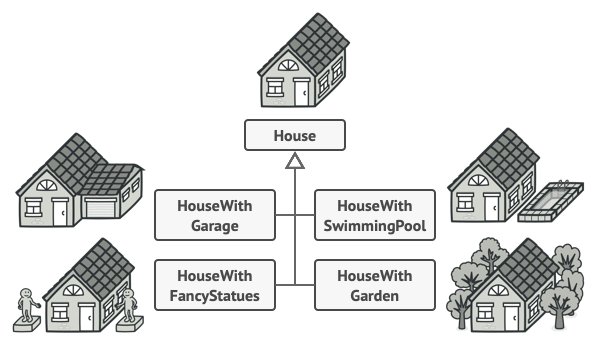
At its core, the Builder Pattern is about breaking down object creation into manageable pieces. Here’s how it works:
- Product: The thing you’re building (e.g., a pizza or a house).
- Builder: A class (or interface) that defines the steps to build the product, like
addCheese()
oraddWalls()
. - Concrete Builder: A specific implementation of the builder that knows how to assemble the parts.
- Director (optional): A class that controls the builder, deciding the order of steps to create specific versions of the product.
This setup lets you create different versions of an object using the same building process—just swap out the builder or tweak the steps.
Why Use the Builder Pattern?
You might be wondering, “Why not just use a constructor?” Well, imagine a House
class with optional features like a garage, pool, or garden. A constructor could look like this:
house = House(True, False, True, False, True, False, ...)
Confusing, right? The Builder Pattern shines when:
- Your object has lots of optional parameters.
- The construction process is complicated and needs clear steps.
- You want to create different flavors of the same object (e.g., a veggie pizza vs. a meat lover’s pizza).
It’s all about clarity and control.
The Algorithm: How It Works
Here’s the step-by-step process to implement the Builder Pattern:
- Define the Product: Create a class for the object you’re building, with all its possible attributes.
- Create a Builder: Make a class with methods for adding each part of the product (e.g.,
addCheese()
,addRoof()
). Chainable methods (returningself
) are a bonus for readability. - Build the Product: Add a
build()
method to return the finished object. - Add a Director (optional): Write a class that uses the builder to assemble the product in a specific way.
- Use It: The client calls the director or builder directly to create the object.
This structure keeps the construction logic separate and reusable.
Simple Example: Building a Pizza
Let’s start with a fun example: building a pizza. We want to add toppings step by step and create different types, like Margherita or Pepperoni.
The Code
# The Product
class Pizza:
def __init__(self):
self.cheese = False
self.pepperoni = False
self.mushrooms = False
def __str__(self):
toppings = []
if self.cheese:
toppings.append("Cheese")
if self.pepperoni:
toppings.append("Pepperoni")
if self.mushrooms:
toppings.append("Mushrooms")
return f"Pizza with {', '.join(toppings)}"
# The Builder
class PizzaBuilder:
def __init__(self):
self.pizza = Pizza()
def add_cheese(self):
self.pizza.cheese = True
return self
def add_pepperoni(self):
self.pizza.pepperoni = True
return self
def add_mushrooms(self):
self.pizza.mushrooms = True
return self
def build(self):
return self.pizza
# The Director
class PizzaDirector:
def make_margherita(self, builder):
return builder.add_cheese().build()
def make_pepperoni(self, builder):
return builder.add_cheese().add_pepperoni().build()
# Usage
builder = PizzaBuilder()
director = PizzaDirector()
margherita = director.make_margherita(builder)
print(margherita) # Output: Pizza with Cheese
pepperoni_pizza = director.make_pepperoni(builder)
print(pepperoni_pizza) # Output: Pizza with Cheese, Pepperoni
What’s Happening?
- The
Pizza
class is the product, tracking toppings. - The
PizzaBuilder
lets you add toppings one by one and returns the final pizza withbuild()
. - The
PizzaDirector
knows how to make specific pizzas by calling the builder’s methods in order.
This makes it super easy to customize pizzas without messy constructors.
Real-World Case: Building a House
Now, let’s scale it up. Imagine you’re building a house with features like walls, a roof, and a garage. The Builder Pattern can handle this complexity gracefully.
The Code
# The Product
class House:
def __init__(self):
self.walls = 0
self.roof = None
self.garage = False
def __str__(self):
return f"House with {self.walls} walls, {self.roof} roof, {'and a garage' if self.garage else 'no garage'}"
# The Builder
class HouseBuilder:
def __init__(self):
self.house = House()
def add_walls(self, count):
self.house.walls = count
return self
def add_roof(self, type):
self.house.roof = type
return self
def add_garage(self):
self.house.garage = True
return self
def build(self):
return self.house
# Usage
builder = HouseBuilder()
house = builder.add_walls(4).add_roof("gable").add_garage().build()
print(house) # Output: House with 4 walls, gable roof, and a garage
Why It’s Useful
In a real project—like a web app for designing homes—you could have multiple builders (e.g., ModernHouseBuilder
, TraditionalHouseBuilder
) to create different styles. The pattern keeps the construction process organized and adaptable.
Advantages and Disadvantages
Advantages
- Step-by-Step Construction: Build complex objects without overwhelming constructors.
- Flexibility: Reuse the same builder for different object versions.
- Readability: Methods like
addGarage()
are way clearer thanTrue, False, True
. - Encapsulation: Hide the messy details of object creation.
Disadvantages
- Extra Classes: You need a builder (and maybe a director), which adds some overhead.
- Not for Simple Cases: If your object has just a couple of fields, this might be overkill.
Use it when complexity calls for it, not for every little object.
Wrapping Up
The Builder Pattern is your go-to tool for creating complex objects cleanly and flexibly. We’ve covered its definition, the concept of separating construction from representation, the algorithm behind it, and two examples: a simple pizza and a real-world house. Plus, you’ve got Python code to play with!
Next time you’re facing a class with a million optional parameters, give the Builder Pattern a shot. It’s a skill that’ll make your code stand out. Stay tuned for our next topic—maybe the Prototype Pattern for cloning objects like a pro. Happy coding, and let me know your thoughts!
Leave a Reply